RemAInders
Flexibility and extensibility are key to integrating new features into digital ecosystem. For example, automatically reminding your colleagues to attend meeting happening this afternoon via an AI.
What Can It Do
Integrate into different parts of the ecosystem — like chatbots, apps, or web services.
Access your reminders anywhere, anytime.
GithubCore Frameworks
- Dotnet Core
- Postgres
- Ef Core
- Jwt
- Rest
and more...
Features
Secured Access
- Utilizes ASP.NET Core Identity to keep track of current users.
- Employs Password authentication for simplified login process.
- Uses encrypted Jwt tokens for secured transactions and access to database.
Seamless AI Integration
- Secure JWT authentication (persistent tokens) enables seamless integration with external services, including AI, for reminder modification.
- Designed to support features like automatic task prioritization
- Effortless integration with scheduling tools or AI agents for managing meeting reminders for attendees.
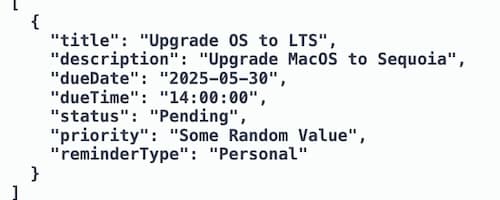
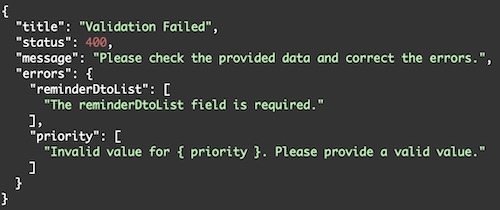
Data Validation
- Validation is performed before modifying data.
- The ModelState filter detects malformed data and invalid input fields.
- Always return detailed response to simplify debugging process.
Implementations
Filters - Model State
1[DefaultValue("My Reminder Title")]
2[Required(ErrorMessage = "{0} is required.")]
3[MaxLength(50, ErrorMessage = "The title must not excess 50 characters long.")]
4public required string Title { get; set; }
5
6[DefaultValue("Describe your reminder")]
7[MaxLength(100, ErrorMessage = "{0} must not exceed {1} characters long.")]
8public string Description { get; set; }
9
10[CustomValidation(typeof(DueDateValidator), nameof(DueDateValidator.ValidateFutureDate))]
11[DefaultValue("2025-04-05")]
12public DateOnly? DueDate { get; set; }
Handles input format and JSON exceptions with short-circuiting before requests reach the controllers.
Middlewares
1catch (SqlException ex)
2{
3 logger.LogError("SQL error occurred: {Exception}", ex.Message);
4 await WriteErrorResponseAsync(
5 context,
6 StatusCodes.Status500InternalServerError,
7 "Database offline. Please try again later."
8 );
9}
10catch (DbUpdateException ex)
11{
12 logger.LogError("Error update database: {Exception}", ex.Message);
13 await WriteErrorResponseAsync(
14 context,
15 StatusCodes.Status500InternalServerError,
16 "Unable to update Database. Please try again later."
17 );
18}
Global Exception Handling
- Handles exception globally (even within controllers) - Reduced nested try blocks.
- Returns relevant message to user when exceptions are triggered.
1builder.Services.AddCors(options =>
2{
3 options.AddPolicy(
4 "DefaultCorsPolicy",
5 builder =>
6 {
7 builder
8 .WithOrigins(ALLOWED_CORS_ORIGINS)
9 .AllowAnyMethod()
10 .AllowAnyHeader()
11 .AllowCredentials();
12 }
13 );
14});
15
16WebApplication app = builder.Build();
17app.UseCors("DefaultCorsPolicy");
18
19app.UseAuthentication();
20app.UseAuthorization();
Access Control and Security Policies
- Configured CORS to manage cross-origin requests, enabling secure frontend integration.
- Authentication and Authorization.
- Implemented rate limiting as a safety precaution.
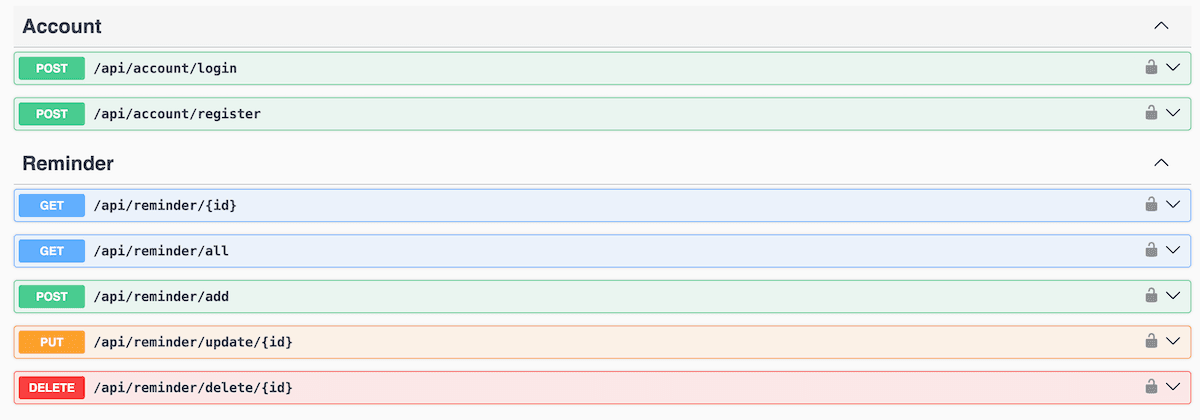
Request Routing & Config.
- UseHttpsRedirection - redirects http to https connection for more security.
- MapControllers routes incoming requests to the appropriate controller actions, enabling easy extension with new features.